Sending emails using Spring mail and Spring boot
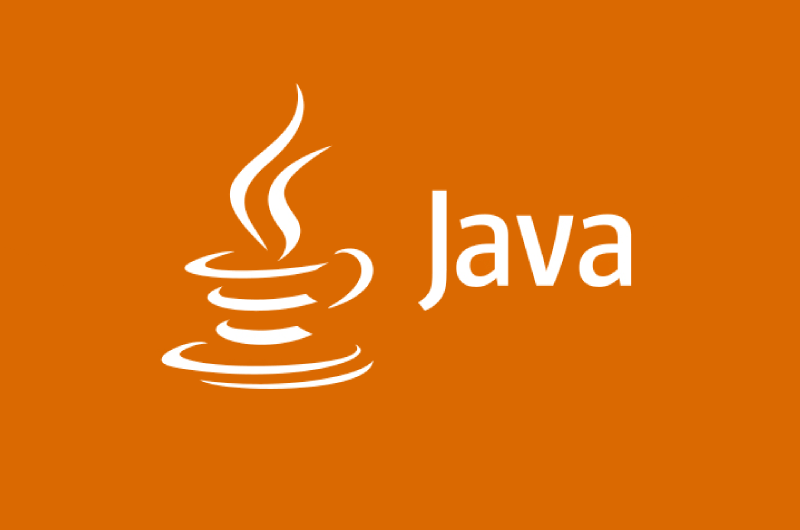
1. Overview
Sending emails is quite a frequent and common task in Java EE projects.
In this article, I will show you how to send emails using Spring mail in a Spring boot application.
2. Implementation
1. Create a new Spring boot project using Spring initializr, then add the spring-boot-starter-mail
dependency to your pom.xml
.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
<version>1.5.10.RELEASE</version>
</dependency>
2. Add the following config properties to your application.properties
.
spring.mail.test-connection=true
spring.mail.host=smtp.gmail.com
spring.mail.port=587
[email protected]
spring.mail.password=password
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
spring.mail.properties.mail.smtp.ssl.trust=smtp.gmail.com
spring.mail.properties.mail.debug=true
For this example, I used the Gmail SMTP server settings, but you can use any SMTP server you want.
3. Create two Java POJOs SimpleMail.java
and HTMLMail.java
to hold mail’s detail.
- SimpleMail.java : we will use it to send a simple text mail.
- HTMLMail.java : we will use it to send an HTML mail.
public class SimpleMail {
private final String to;
public SimpleMail(String to) {
this.to = to;
}
public String getTo() {
return this.to;
}
public String getSubject() {
return "Simple Email Subject";
}
public String getContent() {
return "Hello client,\n This a simple email content !";
}
}
public class HTMLMail {
private final String to;
public HTMLMail(String to) {
this.to = to;
}
public String getTo() {
return this.to;
}
public String getSubject() {
return "HTML Email Subject";
}
public String getContent() {
return "<html>" +
"<body>" +
"<p>Hello client,</p>" +
"<p>This an <strong>HTML</strong> email content !</p>" +
"</body>" +
"</html>";
}
}
4. We will take advantage of the JavaMailSender.java
, to send simple text and HTML emails.
@Service
public class MailSenderService {
@Autowired
private JavaMailSender mailSender;
// Use it to send Simple text emails
public void sendSimpleMail(SimpleMail mail) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(mail.getTo());
message.setSubject(mail.getSubject());
message.setText(mail.getContent());
mailSender.send(message);
}
// Use it to send HTML emails
public void sendHTMLMail(HTMLMail mail) throws MessagingException {
MimeMessage message = mailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, false, "utf-8");
helper.setTo(mail.getTo());
helper.setSubject(mail.getSubject());
message.setContent(mail.getContent(), "text/html");
mailSender.send(message);
}
}
5. Use the created service MailSenderService.java
to send emails via sendSimpleMail(mail)
or sendHTMLMail(mail)
.
@SpringBootApplication
public class SendEmailsSpringBootExampleApplication implements CommandLineRunner {
@Autowired
private MailSenderService senderService;
@Override
public void run(String... args) throws Exception {
// send a simple mail
senderService.sendSimpleMail(new SimpleMail("[email protected]"));
//send an HTML mail
senderService.sendHTMLMail(new HTMLMail("[email protected]"));
}
// ...
}
6. After executing the application, two different emails will be sent to [email protected], simple text email and HTML email.
Find the source code of this example on GitHub.

Software Craftsmanship, Stackextend author and Full Stack developer with 6+ years of experience in Java/Kotlin, Java EE, Angular and Hybris…
I’m Passionate about Microservice architectures, Hexagonal architecture, Event Driven architecture, Event Sourcing and Domain Driven design (DDD)…
Huge fan of Clean Code school, SOLID, GRASP principles, Design Patterns, TDD and BDD.
Nice Article..!
Those who come to read your Information will find lots of helpful and informative tips.