First Step to Lombok Annotations
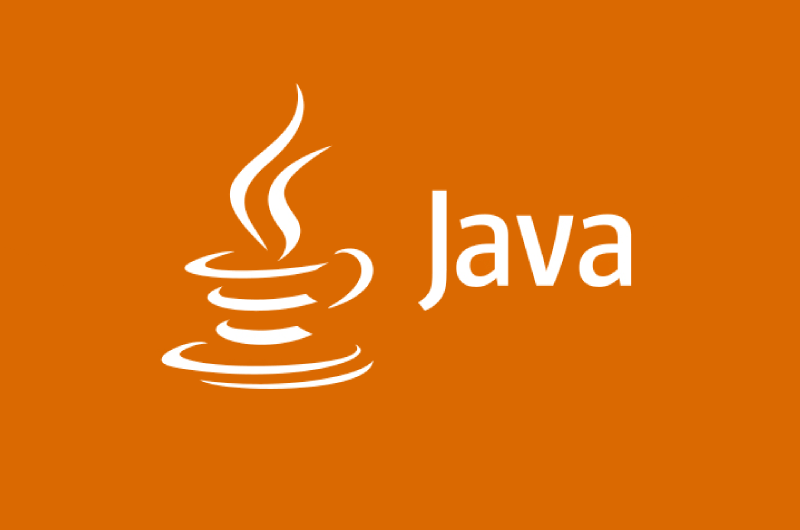
1. Overview
Do you feel bored of writing the same boilerplate and repetitive code in Java again and again ?
So this is what Lombok Project was made for, it helps you to prevent bothering yourself from writing this ugly repetitive code, and instead replace it with some meaningful annotations, so you will end up with a clean and good looking source code. 🙂
In this article, i will show you how to use Lombok with some basic examples.
2. Setup Lombok
1. To be able to use Lombok in your project, add the following dependency to your pom.xml
.
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
<version>1.16.20</version>
</dependency>
2. And add the Lombok plugin to your IDE to be able to deal with Lombok annotations.
- Lombok plugin for IntelliJ IDEA : projectlombok.org/setup/intellij
- Lombok plugin for Eclipse and STS : projectlombok.org/setup/eclipse
Visit Project Lombok official website for other IDEs.
3. Use Lombok
3.1. @NonNull
Adding @NonNull
on the parameter of a method generates a null check statement for these parameter.
With Lombok :
public class NonNullHelloWorld {
public void doSomething(@NonNull String input) {
input.length();
}
}
Without Lombok :
public class NonNullHelloWorld {
public void doSomething(String input) {
if (input == null) {
throw new NullPointerException("input");
}
input.length();
}
}
3.2. @Getter and @Setter
Add @Getter
and/or @Setter
to an attribute to generate getter and setter for it.
With Lombok :
public class GetterSetterHelloWorld {
@Getter @Setter
private String firstAttribute;
@Getter
private String secondAttribute;
}
Without Lombok :
public class GetterSetterHelloWorld {
private String firstAttribute;
private String secondAttribute;
public String getFirstAttribute() { ... }
public void setFirstAttribute(String firstAttribute) { ... }
public String getSecondAttribute() { ... }
}
3.3. @ToString
Use @ToString
to generate under the hood the toString()
method for your classes.
With Lombok :
@ToString
public class ToStringHelloWorld {
private String firstAttribute;
}
Without Lombok :
public class ToStringHelloWorld {
private String firstAttribute;
public String toString() {
return "ToStringHelloWorld(firstAttribute=" + this.firstAttribute + ")";
}
}
There is alsoÂ
@EqualsAndHashCode
that do exactly the same but withequals()
andhashCode()
methods.
3.4. @Data
the @Data
annotation is equivalent to the @Getter
, @Setter
, @ToString
and @EqualsAndHashCode
together, it generates getters, setters, toString, equals and hashCode methods for your classes.
With Lombok :
@Data
public class DataHelloWorld {
private String firstAttribute;
private String secondAttribute;
}
Without Lombok :
public class DataHelloWorld {
private String firstAttribute;
private String secondAttribute;
public String getFirstAttribute() {
//...
}
public String getSecondAttribute() {
//...
}
public void setFirstAttribute(String firstAttribute) {
//...
}
public void setSecondAttribute(String secondAttribute) {
//...
}
public boolean equals(Object o) {
// ...
}
public int hashCode() {
//...
}
public String toString() {
//...
}
}
3.5. @Value
The @Value
is similar to @Data
, the main difference between them is @Value
generates an immutable class.
With Lombok :
@Value
public class ValueHelloWorld {
private String firstAttribute;
private String secondAttribute;
}
Without Lombok :
public final class ValueHelloWorld {
private final String firstAttribute;
private final String secondAttribute;
public ValueHelloWorld(String firstAttribute, String secondAttribute) {
this.firstAttribute = firstAttribute;
this.secondAttribute = secondAttribute;
}
public String getFirstAttribute() {
//...
}
public String getSecondAttribute() {
//...
}
public boolean equals(Object o) {
//...
}
public int hashCode() {
//...
}
public String toString() {
//...
}
}
3.6. @Log and @Slf4j
The @Log
, @Slf4j
and @CommonsLog
annotations add a Logger to your class.
With Lombok :
@Slf4j
public class Slf4jHelloWorld {
public void doSomething() {
log.info("This is a message from Lombok @Slf4j");
}
}
Without Lombok :
public class Slf4jHelloWorld {
private static final Logger log = LoggerFactory.getLogger(Slf4jHelloWorld.class);
public void doSomething() {
log.info("This is a message from Lombok @Slf4j");
}
}
3.7. @Cleanup
Add @Cleanup
to your resources to make sure that they will be cleaned up properly in a finally block.
With Lombok :
public class CleanupHelloWorld {
public void doSomething() throws IOException {
@Cleanup OutputStream out = new FileOutputStream("file");
out.write(1);
out.write(2);
}
}
Without Lombok :
public class CleanupHelloWorld {
public void doSomething() throws IOException {
FileOutputStream out = new FileOutputStream("file");
try {
out.write(1);
out.write(2);
} finally {
if (out != null) {
out.close();
}
}
}
}
You can visit Project Lombok website for more annotations.
Find the source code of this example on GitHub.
4. Conclusion
Project Lombok is a powerful tool that saves time and prevents developers from dealing with boilerplate code.
If for some reason you felt not comfortable with the Lombok ( probably not 🙂 ), you can Delombok your source code to a version without Lombok annotations.

Software Craftsmanship, Stackextend author and Full Stack developer with 6+ years of experience in Java/Kotlin, Java EE, Angular and Hybris…
I’m Passionate about Microservice architectures, Hexagonal architecture, Event Driven architecture, Event Sourcing and Domain Driven design (DDD)…
Huge fan of Clean Code school, SOLID, GRASP principles, Design Patterns, TDD and BDD.
Hi Mouad,
Thank you for this article. Very nice job
I didn’t understand how the generated artifact is a Target.java file, isn’t the byte-code what you wanted to say ?
I other words, when using @Setter @Getter in the top of an attribute, can I do a calls like foo.setAttribute(…) without any compilations errors ?
Thank you in advance Mr Drake 😉
Good remark Saad, my mistake :/
Actually i wanted to do Target.class not Target.java
However thanks to some plugins added to the IDE we can do foo.setAttribute(…) without compilation.
Thank you 😀
Thank you for the explanation Mouad.
Welcome 🙂
Hi Mouad,
Great article about using Lombok. Have you tried using it in a Hybris project? How is your experience.
Hi Raj, Actually integrating Lombok with Hybris should not be hard, however I don’t think we can do much with Lombok in Hybris, because the majority of classes/structures in Hybris are generated.
Hi Mouad,
I tried this but it won’t work for me. I added lombok as an external-dependency in my custom component but the build failed all time and I couldn’t figure it out why. I also have a stackoverflow question about this with more details. If you are interested and maybe have some idea what am I doing wrong then feel free to leave some comment.
Link: https://stackoverflow.com/questions/62305034/lombok-doesnt-generate-getters-in-hybris-custom-component
Thanks!