Index primitive attribute and/or collection in Hybris
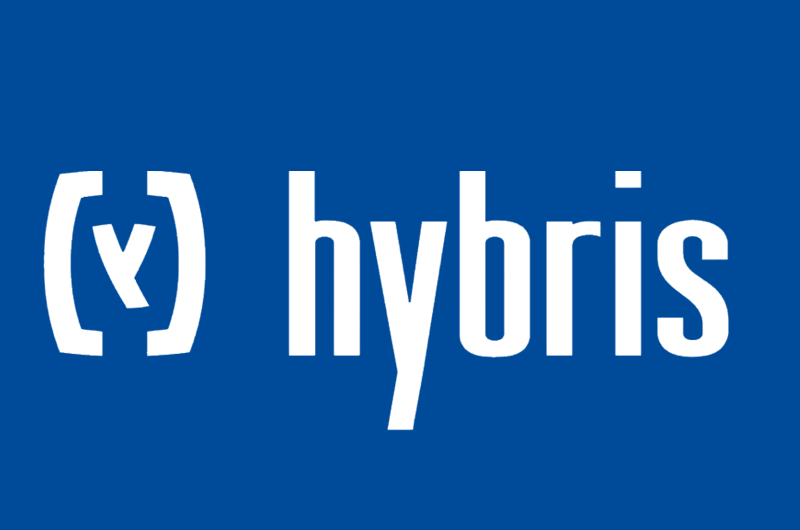
1. Overview
In the last article, we saw how to index a custom attribute to Solr in Hybris using a custom FieldValueProvider
.
In this article, I will show your how to index a primitive attribute (String, int, double…) or collection without the need of creating a custom FieldValueProvider
.
Fortunately, Hybris comes with a pre-existing FieldValueProvider
called SpELValueProvider.java
and registered as springELValueProvider
in Spring.
The idea behind this provider is to make the indexation of primitive attributes and collections easy.
2. Implementation
2.1. Basic primitive attribute
Assuming you want to index a basic Product
attribute of type String
.
public class ProductModel extends ItemModel {
// ...
private String _basicAttribute;
// ...
}
In this case, create a SolrIndexedProperty
with fieldValueProvider
as springELValueProvider
and valueProviderParameter
as basicAttribute
.
INSERT_UPDATE SolrIndexedProperty ; name[unique=true] ;type(code) ;fieldValueProvider ;valueProviderParameter
; basicAttribute ;string ;springELValueProvider ;basicAttribute
The valueProviderParameter takes a Spring Expression Language (SpEL) as value.
2.2. Localized primitive attribute
Indexing a localized attribute is similar to indexing a basic attribute except for the valueProviderParameter
should be like getLocalizedAttribute(#lang)
for a Product attribute called localizedAttribute
.
INSERT_UPDATE SolrIndexedProperty ; name[unique=true] ;type(code) ;localized ;fieldValueProvider ;valueProviderParameter
; localizedAttribute ;string ;true ;springELValueProvider ;getLocalizedAttribute(#lang)
2.3. Basic collection attribute
Assuming you want to index a basic Product
collection attribute of type Collection<String>
.
public class ProductModel {
//...
Collection<String> _basicCollection;
//...
}
In this case, the SolrIndexedProperty
should be as follow.
INSERT_UPDATE SolrIndexedProperty ; name[unique=true] ;type(code) ;multiValue ;fieldValueProvider ;valueProviderParameter
; basicCollection ;string ;true ;springELValueProvider ;basicCollection

Software Craftsmanship, Stackextend author and Full Stack developer with 6+ years of experience in Java/Kotlin, Java EE, Angular and Hybris…
I’m Passionate about Microservice architectures, Hexagonal architecture, Event Driven architecture, Event Sourcing and Domain Driven design (DDD)…
Huge fan of Clean Code school, SOLID, GRASP principles, Design Patterns, TDD and BDD.